Asif M. Python for Geeks. Build production-ready app...2021
- Type:
- Other > E-books
- Files:
- 1
- Size:
- 7.36 MiB (7714049 Bytes)
- Uploaded:
- 2021-10-25 10:20 GMT
- By:
- andryold1
- Seeders:
- 0
- Leechers:
- 0
- Info Hash: 888CDB83C9382DE2AE14637339F3D51190BA8DB1
Textbook in PDF format Take your Python skills to the next level to develop scalable, real-world applications for local as well as cloud deployment Key FeaturesAll code examples have been tested with Python 3.7 and Python 3.8 and are expected to work with any future 3.x releaseLearn how to build modular and object-oriented applications in PythonDiscover how to use advanced Python techniques for the cloud and clustersBook Description Python is a multipurpose language that can be used for multiple use cases. Python for Geeks will teach you how to advance in your career with the help of expert tips and tricks. You'll start by exploring the different ways of using Python optimally, both from the design and implementation point of view. Next, you'll understand the life cycle of a large-scale Python project. As you advance, you'll focus on different ways of creating an elegant design by modularizing a Python project and learn best practices and design patterns for using Python. You'll also discover how to scale out Python beyond a single thread and how to implement multiprocessing and multithreading in Python. In addition to this, you'll understand how you can not only use Python to deploy on a single machine but also use clusters in private as well as in public cloud computing environments. You'll then explore data processing techniques, focus on reusable, scalable data pipelines, and learn how to use these advanced techniques for network automation, serverless functions, and machine learning. Finally, you'll focus on strategizing web development design using the techniques and best practices covered in the book. By the end of this Python book, you'll be able to do some serious Python programming for large-scale complex projects. What you will learnUnderstand how to design and manage complex Python projectsStrategize test-driven development (TDD) in PythonExplore multithreading and multiprogramming in PythonUse Python for data processing with Apache Spark and Google Cloud Platform (GCP)Deploy serverless programs on public clouds such as GCPUse Python to build web applications and application programming interfacesApply Python for network automation and serverless functionsGet to grips with Python for data analysis and machine learningWho this book is for This book is for intermediate-level Python developers in any field who are looking to build their skills to develop and manage large-scale complex projects. Developers who want to create reusable modules and Python libraries and cloud developers building applications for cloud deployment will also find this book useful. Prior experience with Python will help you get the most out of this book. Table of ContentsOptimal Python Development LifecycleUsing Modularization to Elegantly Design Complex ProjectsAdvanced Object Oriented Python ProgrammingPython Libraries for Advanced ProgrammingTesting and Automation with PythonAdvanced Tips and Tricks in PythonMultiprocessing and MultithreadingScaling Out Python to ClustersPython Programming for the CloudUsing Python for Web Development and REST APIUsing Python for micro-services developmentBuilding serverless functions using PythonPython and Machine LearningUsing python for network automation Cover Title Page Copyright and Credits Dedication Contributors Preface Section 1: Python, beyond the Basics Chapter 1: Optimal Python Development Life Cycle Python culture and community Different phases of a Python project Strategizing the development process Iterating through the phases Aiming for MVP first Strategizing development for specialized domains Effectively documenting Python code Python comments Docstring Functional or class-level documentation Developing an effective naming scheme Methods Variables Constant Classes Packages Modules Import conventions Arguments Useful tools Exploring choices for source control What does not belong to the source control repository? Understanding strategies for deploying the code Batch development Python development environments IDLE Sublime Text PyCharm Visual Studio Code PyDev Spyder Summary Questions Further reading Answers Chapter 2: Using Modularization to Handle Complex Projects Technical requirements Introduction to modules and packages Importing modules Using the import statement Using the __import__ statement Using the importlib.import_module statement Absolute versus relative import Loading and initializing a module Standard modules Writing reusable modules Building packages Naming Package initialization file Building a package Accessing packages from any location Sharing a package Building a package as per the PyPA guidelines Installing from the local source code using pip Publishing a package to Test PyPI Installing the package from PyPI Summary Questions Further reading Answers Chapter 3: Advanced Object-Oriented Python Programming Technical requirements Introducing classes and objects Distinguishing between class attributes and instance attributes Using constructors and destructors with classes Distinguishing between class methods and instance methods Special methods Understanding OOP principles Encapsulation of data Encompassing data and actions Hiding information Protecting the data Using traditional getters and setters Using property decorators Extending classes with inheritance Simple inheritance Multiple inheritance Polymorphism Method overloading Method overriding Abstraction Using composition as an alternative design approach Introducing duck typing in Python Learning when not to use OOP in Python Summary Questions Further reading Answers Section 2: Advanced Programming Concepts Chapter 4: Python Libraries for Advanced Programming Technical requirements Introducing Python data containers Strings Lists Tuples Dictionaries Sets Using iterators and generators for data processing Iterators Generators Handling files in Python File operations Using a context manager Operating on multiple files Handling errors and exceptions Working with exceptions in Python Raising exceptions Defining custom exceptions Using the Python logging module Introducing core logging components Working with the logging module What to log and what not to log Summary Questions Further reading Answers Chapter 5: Testing and Automation with Python Technical requirements Understanding various levels of testing Unit testing Integration testing System testing Acceptance testing Working with Python test frameworks Working with the unittest framework Working with the pytest framework Executing TDD Red Green Refactor Introducing automated CI Summary Questions Further reading Answers Chapter 6: Advanced Tips and Tricks in Python Technical requirements Learning advanced tricks for using functions Introducing the counter, itertools, and zip functions for iterative tasks Using filters, mappers, and reducers for data transformations Learning how to build lambda functions Embedding a function within another function Modifying function behavior using decorators Understanding advanced concepts with data structures Embedding a dictionary inside a dictionary Using comprehension Introducing advanced tricks with pandas DataFrame Learning DataFrame operations Learning advanced tricks for a DataFrame object Summary Questions Further reading Answers Section 3: Scaling beyond a Single Thread Chapter 7: Multiprocessing, Multithreading, and Asynchronous Programming Technical requirements Understanding multithreading in Python and its limitations What is a Python blind spot? Learning the key components of multithreaded programming in Python Case study – a multithreaded application to download files from Google Drive Going beyond a single CPU – implementing multiprocessing Creating multiple processes Sharing data between processes Exchanging objects between processes Synchronization between processes Case study – a multiprocessor application to download files from Google Drive Using asynchronous programming for responsive systems Understanding the asyncio module Distributing tasks using queues Case study – asyncio application to download files from Google Drive Summary Questions Further reading Answers Chapter 8: Scaling out Python Using Clusters Technical requirements Learning about the cluster options for parallel processing Hadoop MapReduce Apache Spark Introducing RDDs Learning RDD operations Creating RDD objects Using PySpark for parallel data processing Creating SparkSession and SparkContext programs Exploring PySpark for RDD operations Learning about PySpark DataFrames Introducing PySpark SQL Case studies of using Apache Spark and PySpark Case study 1 – Pi (π) calculator on Apache Spark Case study 2 – Word cloud using PySpark Summary Questions Further reading Answers Chapter 9: Python Programming for the Cloud Technical requirements Learning about the cloud options for Python applications Introducing Python development environments for the cloud Introducing cloud runtime options for Python Building Python web services for cloud deployment Using Google Cloud SDK Using the GCP web console Using Google Cloud Platform for data processing Learning the fundamentals of Apache Beam Introducing Apache Beam pipelines Building pipelines for Cloud Dataflow Summary Questions Further reading Answers Section 4: Using Python for Web, Cloud, and Network Use Cases Chapter 10: Using Python for Web Development and REST API Technical requirements Learning requirements for web development Web frameworks User interface Web server/application server Database Security API Documentation Introducing the Flask framework Building a basic application with routing Handling requests with different HTTP method types Rendering static and dynamic contents Extracting parameters from an HTTP request Interacting with database systems Handling errors and exceptions in web applications Building a REST API Using Flask for a REST API Developing a REST API for database access Case study– Building a web application using the REST API Summary Questions Further reading Answers Chapter 11: Using Python for Microservices Development Technical requirements Introducing microservices Learning best practices for microservices Building microservices-based applications Learning microservice development options in Python Introducing deployment options for microservices Developing a sample microservices-based application Summary Questions Further reading Answers Chapter 12: Building Serverless Functions Using Python Technical requirements Introducing serverless functions Benefits Use cases Understanding the deployment options for serverless functions Learning how to build serverless functions Building an HTTP-based Cloud Function using the GCP Console Case study – building a notification app for cloud storage events Summary Questions Further reading Answers Chapter 13: Python and Machine Learning Technical requirements Introducing machine learning Using Python for machine learning Introducing machine learning libraries in Python Best practices of training data with Python Building and evaluating a machine learning model Learning about an ML model building process Building a sample ML model Evaluating a model using cross-validation and fine tuning hyperparameters Saving an ML model to a file Deploying and predicting an ML model on GCP Cloud Summary Questions Further reading Answers Chapter 14: Using Python for Network Automation Technical requirements Introducing network automation Merits and challenges of network automation Use cases Interacting with network devices Protocols for interacting with network devices Interacting with network devices using SSH-based Python libraries Interacting with network devices using NETCONF Integrating with network management systems Using location services endpoints Getting an authentication token Getting network devices and an interface inventory Updating the network device port Integrating with event-driven systems Creating subscriptions for Apache Kafka Processing events from Apache Kafka Renewing and deleting a subscription Summary Questions Further reading Answers About Packt Other Books You May Enjoy Index
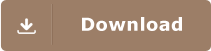